- nnenna hacks
- Posts
- Practice Problem Solving with JavaScript
Practice Problem Solving with JavaScript
Practice coding in JavaScript by breaking down a problem, researching, & refactoring

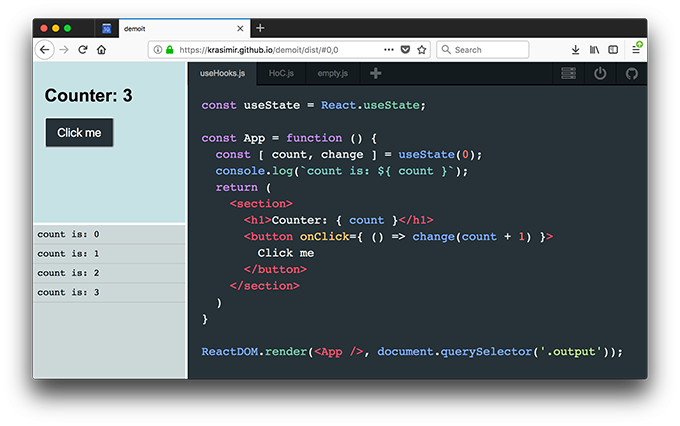
Someone in my network recently reached out to get help with writing algorithms with JavaScript. I was delighted to be a resource in any way possible, although I didn’t know exactly what that would look like in practice.
Interviews are hard. Coding while problem solving and talking through a solution can be an intense process that’s nerve-wrecking and draining. But having a general process for approaching the problem can help steer you in the right direction to solve it.
She sent me an algorithm prompt and asked how I would’ve written the solution for it. But I didn’t want to simply send my solution to her. There’s more that goes into that process than just writing the code. There’s also:
figuring out and explaining how to break down a problem into comprehensible steps
refactoring to improve the logic and readability when possible
if possible, leveraging online resources like a developer would on the job to solve parts of a problem
So let me break down exactly how this problem can be solved in a real world sense!
Background
There are many ways to write a function. I’m simply choosing one particular way to approach it and write it in JavaScript!
Here are the instructions:
Given a list of integers, create a function that returns the highest product between three of those numbers. For example, given the array [1, 10, 2, 6, 5, 3]
the highest product would 10 * 6 * 5 = 300
Before we dive into the code, let’s leverage an online JavaScript editor to write and run everything as we go along. I’m going to be using the Progamiz JavaScript Online Compiler for this!

Screenshot — Programiz.com
Feel free to copy and paste the above instructions into your online compiler as a comment so you can easily refer back to the instructions as we’re writing the code.
Now, let’s break down the problem and figure out how to solve it.
Breaking Down the Problem
The instructions want us to create a function that will be able to take in an array as an argument and return the multiplication of the highest three numbers in the array.
I break this down into steps, which can be used as a guide for what to write next in the function that’ll do all the magic.
How can you grab three of the highest numbers in an array? How do we determine which elements are the highest?
One idea that comes to mind is to order the array from greatest to least. That way, after sorting, the three highest numbers will always be the first three elements in the array. Then we extract those first three elements and do some multiplication on them to get our answer.
Let’s scaffold the function with what we think we need to do so far. I’m going to name the function highestProduct
and I’ll name the input parameter inputArray
.
function highestProduct(inputArray) {
// sort the numbers array in order from highest to lowest
// take the first three numbers in the array
// multiply the three numbers
// return the product
}const exampleArray = [1, 10, 2, 6, 5, 3];
console.log(highestProduct(exampleArray));
Below the function, I use the array from the instruction example as the input to test that the function will work. We’ve got our steps as comments in the code. Let’s tackle the first step.
When we invoke highestProduct
, we want the exampleArray
to be properly sorted from highest to lowest numbers, since it doesn’t start off that way. How do we do that in JavaScript? Let’s Google it.
I searched “sort highest to lowest javascript” and found this result:
// Sort an array of numbers
let numbers = [5, 13, 1, 44, 32, 15, 500]//Highest to lowest
let highestToLowest = numbers.sort((a, b) => b-a);
//Output: [500,44,32,15,13,5,1]
This Google search result looks like exactly what we want to achieve as a first step in our own function. We can apply that sort
method to the inputArray
// sort the numbers array in order from highest to lowest
inputArray.sort((a, b) => b-a);
If you console.log
the inputArray
after that line of code and run the JavaScript compiler, it should print out the output that is now a sorted version of the array, which is [ 10, 6, 5, 3, 2, 1 ]
.
This leads us to the next step. How do we grab the first three numbers of the array? There are a few ways this can be done. One way is to directly reference the elements via their index values like this: inputArray[0]
And to multiply the first three numbers, we’d do this:
inputArray[0] * inputArray[1] * inputArray[2]
and return that value in the function to finish solving the problem! This is how it’d look:
function highestProduct(inputArray) {
// sort the numbers array in order from highest to lowest
inputArray.sort((a, b) => b-a); // take the first three numbers in the array
// multiply the three numbers
const product = inputArray[0] * inputArray[1] * inputArray[2];
// return the product
return product;
}const exampleArray = [1, 10, 2, 6, 5, 3];
console.log(highestProduct(exampleArray));
Testing Your Input and Reviewing the Output
Ideally, we’d be able to continuously invoke the function with different arrays and get the correct outcome. By modifying the exampleArray
to play around with different lists of numbers, you should be able to still get the product of the three highest numbers from the input.
Doing this is helpful in case there are errors you unexpectedly run into when re-running the compiler. It also can inspire you to think about the what if’s with the type of data you plug into the function. From that, we can assess ways to make improvements to the code.
Iterate on Your Solution
After you’ve written the function that works and played around with different example arrays to test that your output is correct, there’s another phase in problem-solving that is crucial to consider, whether for practice or when you’re on the job. Here are the questions to ask yourself:
Can this be improved in any way?
Am I making any assumptions that would need to be accounted for in the code?
Leveraging More JavaScript Built-In Methods
One way to refactor the code could be to leverage more built-in JavaScript methods that’d make the function logic a bit more sophisticated, like the way we were able to use the sort
method.
There are methods for grabbing only specific elements in an array (in our case, we want the three highest) and multiplying them by each other.
After doing a bit of research in the MDN web docs related to built-in Array methods, I found that some methods we could use are slice and reduce.
We can use slice()
to pluck out the first three elements in our array and reduce()
to accumulate multiplication over the three elements. Here’s how that could look:
const threeHighest = inputArray.slice(0,3);
const product = threeHighest.reduce((previousNum, currentNum) => previousNum * currentNum);
Checking for Edge Cases
In our scenario, I think there are definitely more opportunities for refactoring. One assumption is that any array we choose to plug into highestProduct
will include enough elements in the array to return the product of the three highest numbers. What if the array had only 2 elements?
Testing it out now, here’s what the output would be:
const invalidArray = [4,9];console.log(highestProduct(invalidArray))// Output: NaN
If we want to handle a case for too few elements in an array, we could add in conditional logic in the function so that the function does not proceed with attempting to extract the three elements we would need for the multiplication step. That could look something like this:
if (inputArray.length >= 3) { rest of the logic here ... } return 'Input error';
How you decide to approach this step could look different and many solutions would suffice, but the main takeaway there is that we are explicitly accounting for arrays that are too short in length for the conditions of the problem we’re aiming to solve.
Another assumption is that every element in the array will be a number and not another data type that could result in an error. We might get a similar NaN
output if our example array was [10, 'hello', 5, 45];
So let’s check the elements in the array and clean it at the beginning of the function to handle this edge case. If we get other data types like strings in our input array, we could kick those elements out and continue with the elements that are, in fact, numbers. Two methods we could do that with are filter()
and isNaN()
. I found isNaN
by searching in Google “check if number javascript”. Here’s the resource I found for that and how I applied the two methods to the inputArray
.
// clean the data if there are elements that are not numbers
let numbersArray = inputArray.filter(element => !isNaN(element));
Here’s the highestProduct
function with the latest refactor:
function highestProduct(inputArray) { // clean the data if there are elements that are not numbers
let numbersArray = inputArray.filter(element => !isNaN(element)); // check if array has enough elements
if (numbersArray.length >= 3) { // sort the numbers from highest to lowest
numbersArray.sort((a, b) => b-a);
// take only the first three elements
const threeHighest = numbersArray.slice(0,3);
// multiply the three elements
const product = threeHighest.reduce(
(previousNum, currentNum) => previousNum * currentNum);
// return the product
return product;
}
return 'The input does not meet the criteria';
}
Google is Your Friend
When possible, targeted Google searches can help you write certain parts of your code. The biggest aspect of this is knowing how to word your search to lead you to the most helpful information available online. The other part of this is interpreting the results you peruse to see what part of it could be useful and applicable to the specific problem you’re working through.
Some more helpful practice would be to write out your own version of these built-in methods for algorithm practice!
With more experience, as many developers know, the better you’ll become at determining what information is useful to you and what you can throw out. And the more you familiarize yourself with the methods and properties available in JavaScript, the easier it’ll become to memorize the proper syntax you’ll need for writing code in the future.
I like to think of Google search as an asset to your skill set development, not as a replacement for your own instinct.
I enjoy talking about software engineering, fitness, productivity, DevRel, and self-improvement! Feel free to find me on LinkedIn and BlueSky.
Happy coding! :)
Reply